Engineering in computer science is the discipline of designing, developing, and maintaining software systems. It encompasses a wide range of topics, including software design, software development, software testing, and software maintenance. Engineering in computer science is essential for the development of new and innovative software applications, and it plays a vital role in the functioning of modern society.
Engineering in computer science is important because it helps to ensure that software systems are reliable, efficient, and secure. It also helps to reduce the cost of software development and maintenance. Engineering in computer science has a long and rich history, and it has been instrumental in the development of many of the technologies that we rely on today.
The main topics covered in engineering in computer science include:
- Software design
- Software development
- Software testing
- Software maintenance
Engineering in Computer Science
Engineering in computer science is a vast and complex field, encompassing a wide range of topics and specializations. However, there are some key aspects that are essential to understanding the discipline as a whole.
- Software design: The process of creating a blueprint for a software system, defining its structure, components, and behavior.
- Software development: The process of writing and testing code to implement a software design.
- Software testing: The process of evaluating a software system to ensure that it meets its requirements and is free of defects.
- Software maintenance: The process of keeping a software system up-to-date, fixing bugs, and adding new features.
- Algorithms: A set of instructions that a computer follows to perform a task.
- Data structures: A way of organizing and storing data in a computer so that it can be efficiently accessed and processed.
- Computer architecture: The design of a computer system, including its hardware and software components.
- Operating systems: The software that manages the hardware and software resources of a computer.
These are just a few of the key aspects of engineering in computer science. By understanding these concepts, you will be well on your way to understanding the discipline as a whole.
Software design
Software design is a critical component of engineering in computer science. It is the process of creating a blueprint for a software system, defining its structure, components, and behavior. This blueprint is used by developers to implement the system and by testers to verify that it meets its requirements.
Software design is important because it helps to ensure that software systems are reliable, efficient, and secure. It also helps to reduce the cost of software development and maintenance. For example, a well-designed software system will be easier to understand and modify, which can save time and money in the long run.
There are many different software design methodologies, each with its own strengths and weaknesses. Some of the most common methodologies include:
- Object-oriented design: This methodology focuses on creating software systems that are composed of objects. Objects are self-contained entities that can interact with each other.
- Structured design: This methodology focuses on creating software systems that are composed of modules. Modules are independent units of code that can be combined to create larger systems.
- Component-based design: This methodology focuses on creating software systems that are composed of components. Components are pre-built, reusable software units that can be combined to create larger systems.
The choice of software design methodology depends on the specific needs of the project. However, all software design methodologies share a common goal: to create software systems that are reliable, efficient, secure, and easy to maintain.
Software development
Software development is a critical part of engineering in computer science. It is the process of writing and testing code to implement a software design. This code is what makes the software system work, and it must be carefully written and tested to ensure that it is reliable, efficient, and secure.
- Coding: The process of writing code to implement a software design. This code is typically written in a programming language, such as Java, C++, or Python.
- Testing: The process of evaluating code to ensure that it meets its requirements and is free of defects. This testing can be done manually or automatically, and it is essential for ensuring that the software system is reliable.
- Debugging: The process of finding and fixing defects in code. This can be a challenging task, but it is essential for ensuring that the software system is stable and reliable.
- Documentation: The process of creating documentation for a software system. This documentation can include user manuals, technical specifications, and design documents. It is essential for ensuring that the software system is easy to understand and maintain.
Software development is a complex and challenging process, but it is essential for creating high-quality software systems. By understanding the different aspects of software development, you can be better prepared to contribute to the development of successful software systems.
Software testing
Software testing is a critical component of engineering in computer science. It is the process of evaluating a software system to ensure that it meets its requirements and is free of defects. This process is essential for ensuring that software systems are reliable, efficient, and secure.
- Verification and validation: Software testing can be divided into two main categories: verification and validation. Verification ensures that the software system meets its specifications, while validation ensures that the software system meets the needs of its users.
- Types of testing: There are many different types of software testing, each with its own purpose. Some of the most common types of testing include unit testing, integration testing, system testing, and acceptance testing.
- Test automation: Test automation is the use of software tools to automate the testing process. This can save time and money, and it can also improve the accuracy and reliability of testing.
- Importance of software testing: Software testing is an essential part of engineering in computer science. It helps to ensure that software systems are reliable, efficient, and secure. It also helps to reduce the cost of software development and maintenance.
By understanding the importance of software testing and the different types of testing available, you can be better prepared to contribute to the development of high-quality software systems.
Software maintenance
Software maintenance is a critical component of engineering in computer science. It is the process of keeping a software system up-to-date, fixing bugs, and adding new features. This process is essential for ensuring that software systems are reliable, efficient, and secure. Without proper maintenance, software systems can become outdated, buggy, and vulnerable to security breaches.
There are many different types of software maintenance activities, including:
- Corrective maintenance: This type of maintenance involves fixing bugs in the software system.
- Adaptive maintenance: This type of maintenance involves modifying the software system to meet new requirements.
- Perfective maintenance: This type of maintenance involves improving the performance or quality of the software system.
- Preventive maintenance: This type of maintenance involves performing regular maintenance tasks to prevent problems from occurring.
Software maintenance is an important part of the software development lifecycle. It helps to ensure that software systems are reliable, efficient, and secure. It also helps to reduce the cost of software development and maintenance. For example, a well-maintained software system will be easier to update and modify, which can save time and money in the long run.
By understanding the importance of software maintenance and the different types of maintenance activities, you can be better prepared to contribute to the development and maintenance of high-quality software systems.
Algorithms
Algorithms are a fundamental component of engineering in computer science. They are the step-by-step instructions that a computer follows to perform a task. Algorithms are used in a wide variety of applications, from simple tasks like sorting a list of numbers to complex tasks like playing chess.
Algorithms are important because they allow computers to solve problems efficiently. Without algorithms, computers would be much slower and less efficient at performing tasks.
For example, consider the task of sorting a list of numbers. One simple algorithm for sorting a list of numbers is the bubble sort algorithm. The bubble sort algorithm compares each pair of adjacent numbers in the list and swaps them if they are out of order. This process is repeated until the list is sorted.
The bubble sort algorithm is a simple and efficient algorithm for sorting a small list of numbers. However, it is not the most efficient algorithm for sorting large lists of numbers. There are more efficient algorithms, such as the quicksort algorithm, that can be used to sort large lists of numbers much faster than the bubble sort algorithm.
The choice of algorithm for a particular task depends on a number of factors, including the size of the input data, the desired time complexity, and the desired space complexity. By understanding the different types of algorithms and their performance characteristics, computer scientists can choose the best algorithm for each task.
Data structures
Data structures are a fundamental component of engineering in computer science. They are the way that data is organized and stored in a computer so that it can be efficiently accessed and processed. Data structures are used in a wide variety of applications, from simple tasks like storing a list of names to complex tasks like representing a graph of relationships between objects.
The choice of data structure for a particular application depends on a number of factors, including the type of data being stored, the operations that will be performed on the data, and the performance requirements of the application. For example, a simple array data structure can be used to store a list of names, while a more complex tree data structure can be used to represent a graph of relationships between objects.
Data structures are an important part of engineering in computer science because they allow computers to store and process data efficiently. Without data structures, computers would be much slower and less efficient at performing tasks.
Here are some real-life examples of how data structures are used in engineering in computer science:
- Databases use data structures to store and organize data in a way that makes it easy to access and retrieve.
- Compilers use data structures to represent the source code of a program and to generate the machine code that the computer can execute.
- Operating systems use data structures to manage memory, processes, and other resources.
By understanding the different types of data structures and their performance characteristics, computer scientists can choose the best data structure for each application. This understanding is essential for developing efficient and effective software systems.
Computer architecture
Computer architecture is the design of a computer system, including its hardware and software components. It is a fundamental component of engineering in computer science, as it determines the performance, efficiency, and reliability of a computer system. Without a sound understanding of computer architecture, it is impossible to design and build high-quality computer systems.
Computer architecture is closely related to engineering in computer science in several ways. First, computer architecture provides the foundation for the design and implementation of operating systems, programming languages, and other software components. Second, computer architecture influences the performance and efficiency of algorithms and data structures. Third, computer architecture is essential for understanding the security and reliability of computer systems.
There are many real-life examples of the importance of computer architecture. For example, the design of the central processing unit (CPU) is critical to the performance of a computer system. The CPU is responsible for executing instructions, and the design of the CPU determines how quickly and efficiently instructions can be executed. Another example is the design of the memory hierarchy. The memory hierarchy is responsible for storing data and instructions, and the design of the memory hierarchy determines how quickly and efficiently data and instructions can be accessed.
Understanding computer architecture is essential for computer scientists and engineers. This understanding allows computer scientists and engineers to design and build high-quality computer systems that meet the needs of users. It also allows computer scientists and engineers to develop new and innovative computer architectures that push the boundaries of what is possible.
Operating systems
Operating systems are an essential component of engineering in computer science. They provide the foundation for all other software applications, and they play a critical role in managing the hardware and software resources of a computer. Without an operating system, a computer would be nothing more than a collection of electronic components.
Operating systems perform a wide range of tasks, including:
- Managing memory and storage
- Scheduling processes and threads
- Providing input and output services
- Managing security and permissions
Operating systems are complex pieces of software, and they must be carefully designed and implemented in order to ensure the efficient and reliable operation of a computer system. Engineering in computer science provides the knowledge and skills necessary to design and build high-quality operating systems.
There are many different types of operating systems, each with its own strengths and weaknesses. Some of the most popular operating systems include Windows, macOS, and Linux. The choice of operating system for a particular computer system depends on a number of factors, including the type of hardware, the intended use of the computer, and the user’s preferences.
Understanding operating systems is essential for computer scientists and engineers. This understanding allows computer scientists and engineers to design and build high-quality computer systems that meet the needs of users. It also allows computer scientists and engineers to develop new and innovative operating systems that push the boundaries of what is possible.
FAQs about Engineering in Computer Science
Engineering in computer science is a rapidly growing field that offers many exciting opportunities for those interested in technology. However, there are also many common misconceptions about engineering in computer science, which can make it difficult for students to decide if it is the right field for them.
Here are answers to some of the most frequently asked questions about engineering in computer science:
Question 1: What is engineering in computer science?
Engineering in computer science is the application of engineering principles to the design, development, and maintenance of software systems. Computer science engineers use their knowledge of mathematics, science, and engineering to solve problems and create new technologies.
Question 2: What are the different types of engineering in computer science?
There are many different types of engineering in computer science, including:
- Software engineering
- Computer hardware engineering
- Computer network engineering
- Data science
- Artificial intelligence
Question 3: What are the benefits of studying engineering in computer science?
There are many benefits to studying engineering in computer science, including:
- High earning potential
- Job security
- Opportunities to work on cutting-edge technologies
- Opportunities to make a difference in the world
Question 4: What are the challenges of studying engineering in computer science?
There are some challenges to studying engineering in computer science, including:
- The coursework can be difficult
- The field is constantly changing
- It can be difficult to find a job in a specific area of interest
Question 5: Is engineering in computer science the right field for me?
Engineering in computer science is a great field for people who are interested in technology and who are good at math and science. If you are looking for a challenging and rewarding career, then engineering in computer science may be the right field for you.
Question 6: What are the future prospects for engineering in computer science?
The future prospects for engineering in computer science are excellent. The demand for computer science engineers is expected to grow in the coming years as businesses and governments increasingly rely on technology.
Overall, engineering in computer science is a rewarding field with many opportunities for growth. If you are interested in technology and are good at math and science, then engineering in computer science may be the right field for you.
Transition to the next article section:
Now that you have learned more about engineering in computer science, you can explore some of the other articles in this section to learn more about specific topics in engineering in computer science.
Tips for Engineering in Computer Science
Engineering in computer science is a rapidly growing field that offers many exciting opportunities for those interested in technology. However, it can also be a challenging field, and it is important to be prepared for the challenges that you will face. Here are five tips to help you succeed in engineering in computer science:
1. Get a strong foundation in mathematics and science.
Mathematics and science are the foundation of engineering in computer science. You will need to have a strong understanding of these subjects in order to succeed in your coursework and in your career.
2. Develop strong problem-solving skills.
Engineering in computer science is all about solving problems. You will need to be able to think critically and creatively in order to come up with solutions to complex problems.
3. Be prepared to work hard.
Engineering in computer science is a demanding field. You will need to be prepared to put in long hours of study and work in order to succeed.
4. Get involved in extracurricular activities.
Extracurricular activities can help you to develop the skills that you need to succeed in engineering in computer science. There are many different extracurricular activities available, such as coding clubs, robotics clubs, and math clubs.
5. Seek out mentors.
Mentors can provide you with guidance and support as you pursue your engineering in computer science degree. Mentors can be found in a variety of places, such as your professors, your classmates, and your industry contacts.
By following these tips, you can increase your chances of success in engineering in computer science.
Transition to the article’s conclusion:
Engineering in computer science is a rewarding field that offers many opportunities for growth. By following these tips, you can increase your chances of success in this challenging and exciting field.
Conclusion
Engineering in computer science is a rapidly growing and dynamic field that is constantly evolving. As technology advances, so too does the need for skilled engineers who can design, develop, and maintain the software systems that power our world.
This article has explored the many different aspects of engineering in computer science, from the fundamental concepts of software design and development to the more complex topics of computer architecture and operating systems. We have also discussed the benefits and challenges of studying engineering in computer science, and provided some tips for students who are interested in pursuing this field.
Engineering in computer science is a challenging but rewarding field that offers many opportunities for growth. If you are interested in technology and are passionate about solving problems, then engineering in computer science may be the right field for you.
Youtube Video:
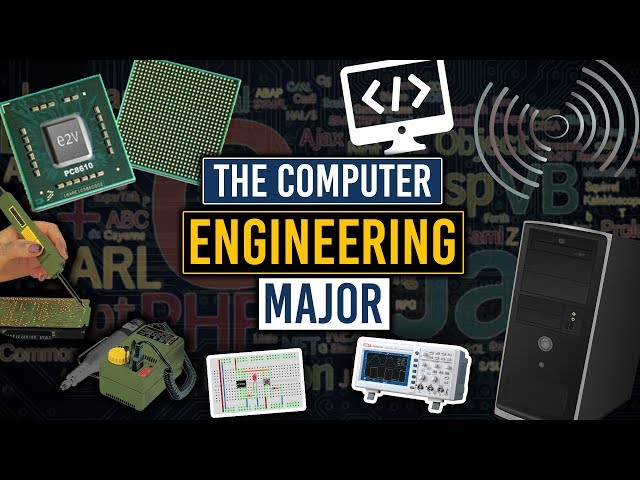